Garbage Collection in Java
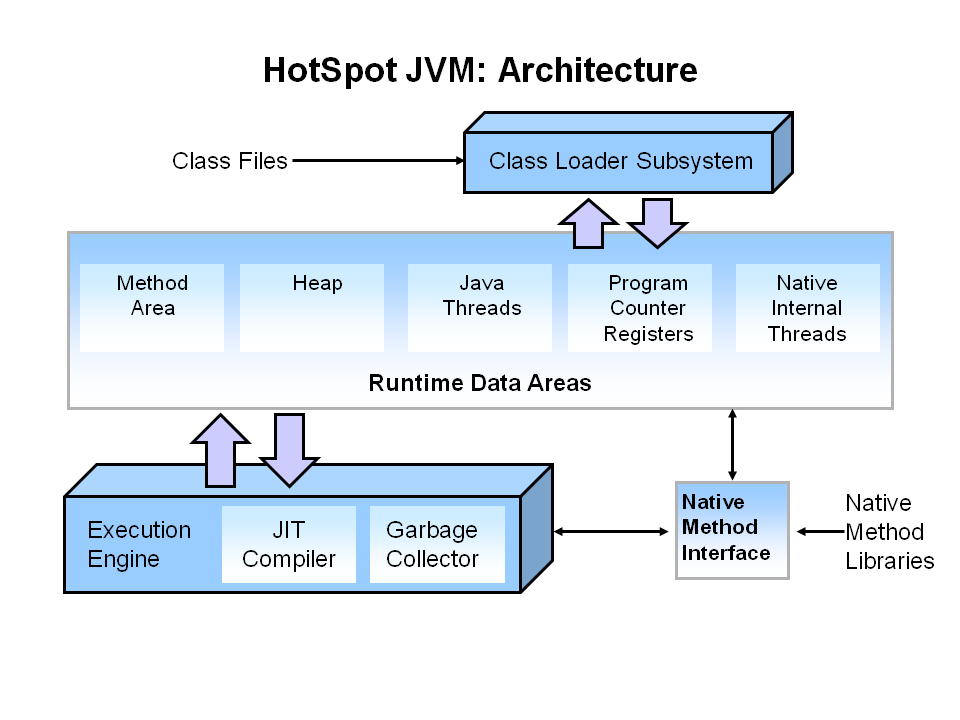
Introduction to Garbage Collection in Java
Garbage Collection (GC) in Java is an essential feature of the Java Virtual Machine (JVM) that helps manage memory automatically. It allows Java applications to reclaim memory by identifying and disposing of objects that are no longer in use, freeing up memory for new objects. This process helps prevent memory leaks, which could otherwise lead to out-of-memory errors and degraded application performance.
Unlike languages like C and C++, where developers manually allocate and deallocate memory, Java’s garbage collector automatically handles this process. This automation is crucial for Java’s memory management, as it reduces the risk of memory-related bugs and makes Java a safer and more accessible language for developers.
Why is Garbage Collection Important in Java?
- Automatic Memory Management: By automating memory allocation and deallocation, Java reduces the risk of memory leaks and makes the code simpler and more maintainable.
- Improved Performance: Effective garbage collection can improve application performance by optimizing memory usage and preventing the system from running out of memory.
- Safety: With garbage collection, Java applications are less prone to memory-related errors like dangling pointers, which are common in languages where memory is managed manually.
How Does Java Garbage Collection Work?
Java’s garbage collector operates within the JVM heap, where objects are allocated memory when created. The garbage collector follows a set of algorithms to determine which objects are no longer reachable or in use. When it finds these “unreachable” objects, it reclaims their memory, making it available for new objects.
The key steps include:
- Identification: The GC identifies objects that are no longer in use.
- Marking: It marks these objects as candidates for removal.
- Sweeping: Finally, it deallocates memory occupied by these marked objects, which can then be reused.
The Basics of Reachability in Java Garbage Collection
The GC determines an object’s eligibility for collection based on its reachability. An object is eligible for collection if it is no longer reachable from any live thread or static reference. Reachability is defined as follows:
- Strong Reachability: Objects that are strongly reachable cannot be collected, as they are directly referenced.
- Soft, Weak, and Phantom Reachability: These types of references allow more flexibility, where the garbage collector can reclaim memory based on certain conditions (e.g., when memory is low or when no strong references are left).
Types of Garbage Collectors in Java
The JVM provides several garbage collection algorithms optimized for different needs. These include:
- Serial GC: Single-threaded, suitable for small applications.
- Parallel GC: Uses multiple threads and is ideal for high-throughput applications.
- CMS (Concurrent Mark-Sweep) GC: Designed for low-latency applications, reducing pause times.
- G1 (Garbage-First) GC: Focuses on predictable pause times and is efficient for large heaps.
- ZGC and Shenandoah: Designed for low-pause times in large-memory applications, ideal for real-time and interactive applications.
To make an object eligible for garbage collection in Java, you need to ensure that there are no live references pointing to it. Here’s how to achieve that and how to explicitly request garbage collection:
1. Making an Object Eligible for Garbage Collection
Java automatically garbage collects objects that are no longer reachable, meaning there are no references to them in the program. Here are a few ways to make an object eligible for garbage collection:
Nullifying References
- Set the reference variable to
null
, which removes the reference to the object, making it eligible for garbage collection.
java
Copy code
MyClass myObject = new MyClass(); // Use the object myObject = null; // Now eligible for GC
Reassigning References
- If a reference is reassigned to point to another object, the previous object (if not referenced elsewhere) becomes eligible for garbage collection.
java
Copy code
MyClass myObject = new MyClass(); myObject = new MyClass(); // The first object is now eligible for GC
Using Local Variables (Scope-Based Collection)
- Objects created within a method become unreachable once the method execution completes, making them eligible for GC if no references exist outside.
java
Copy code
public void myMethod() { MyClass myObject = new MyClass(); // Once myMethod completes, myObject is eligible for GC }
Using Weak References
- In scenarios where you want an object to be garbage-collected if no strong references exist, you can use a
WeakReference
.
java
Copy code
WeakReference<MyClass> weakRef = new WeakReference<>(new MyClass()); // If no strong references, this object can be collected
2. Explicitly Requesting Garbage Collection
While Java’s garbage collector (GC) is managed by the JVM, you can request it to run explicitly. However, keep in mind that this is only a request—the JVM may choose to ignore it. Here’s how:
Using System.gc()
- The
System.gc()
method suggests that the JVM should make an effort to perform garbage collection. It doesn’t guarantee immediate collection but is a common way to request it.
java
Copy code
System.gc();
Using Runtime.getRuntime().gc()
- Another way to request garbage collection is through the
Runtime
class.
java
Copy code
Runtime.getRuntime().gc();
When to Use Explicit GC Calls (with Caution)
Explicit garbage collection requests are rarely needed in modern Java applications, as the JVM is highly optimized to handle GC automatically. However, there are rare cases where invoking System.gc()
might be appropriate:
- Memory-Intensive Applications: If your application has very specific memory constraints or must release memory before a known intensive operation.
- Testing and Benchmarking: To force garbage collection between tests or benchmark runs to ensure each test starts with a fresh heap state.
In Java, there are two primary ways to suggest the JVM run garbage collection:
- Using
System.gc()
- Using
Runtime.getRuntime().gc()
Both methods essentially do the same thing and are interpreted by the JVM as a suggestion to run garbage collection. However, neither guarantees that the garbage collector will run immediately or at all; it’s up to the JVM’s discretion. Here’s a look at each approach and some best practices around them.
1. Using System.gc()
The System.gc()
method is the most commonly used approach. It’s a static method, meaning it’s straightforward to call without creating any additional Runtime
instances.
java
Copy code
System.gc();
2. Using Runtime.getRuntime().gc()
Runtime.getRuntime().gc()
is an alternative way to request garbage collection. Here, getRuntime()
is called on Runtime
, which returns the current runtime instance, and then gc()
is invoked on it.
java
Copy code
Runtime.getRuntime().gc();
Comparison: Which One to Use?
Since both approaches effectively request the same behavior from the JVM, they’re equally valid in terms of functionality. However, System.gc()
is typically the preferred method because:
- Simplicity:
System.gc()
is a static call and is easier to use, whileRuntime.getRuntime().gc()
requires an extra method call (getRuntime()
). - Readability:
System.gc()
is immediately recognizable and explicitly suggests garbage collection.
When to Use Explicit GC Calls
Explicit calls to garbage collection (System.gc()
or Runtime.getRuntime().gc()
) are generally not recommended for most applications. Java’s garbage collector is designed to be automatic and optimized, and in most cases, it’s better to let the JVM manage memory. However, explicit GC requests may be helpful in specific situations:
- Memory-Intensive Operations: If an application performs high-memory operations, you might consider calling
System.gc()
after a known memory-intensive task to reclaim memory before the next heavy operation. - Testing and Benchmarking: For testing and benchmarking purposes, explicitly requesting GC can ensure that each test run begins with a “clean” heap state, making the results more consistent.
- Application Shutdown: In applications where you need to release resources explicitly during shutdown, a call to
System.gc()
may be acceptable to ensure cleanup before termination.
Key Takeaway
- Best Practice: Prefer
System.gc()
overRuntime.getRuntime().gc()
for readability and simplicity. - Recommendation: Use these calls sparingly, if at all. In most cases, let the JVM decide when to perform garbage collection, as it has internal algorithms to optimize performance based on the application’s behavior.
The JVM invokes the garbage collector automatically under several conditions to free up memory by removing objects that are no longer reachable or referenced by the application. Here are the primary scenarios when the garbage collector may be triggered:
- Memory Threshold is Reached: When the JVM notices that available heap memory is low, it may trigger garbage collection to reclaim space. This typically happens when memory usage reaches a certain threshold, which can vary based on the garbage collection algorithm in use and the configuration.
- Explicit Request: Although you can request garbage collection explicitly with
System.gc()
orRuntime.getRuntime().gc()
, it’s merely a suggestion to the JVM. The JVM might ignore this call, as there’s no guarantee that calling these methods will actually trigger garbage collection. - Minor Garbage Collection (Young Generation): When the young generation (Eden space and Survivor spaces) is filled with objects, a “minor” garbage collection is triggered. This type of collection is faster and occurs more frequently since the young generation is usually where short-lived objects are stored.
- Major Garbage Collection (Old Generation): When objects survive minor garbage collections and move to the old generation, and the old generation space starts filling up, a “major” garbage collection occurs. This is typically more intensive and takes longer, as it collects objects from the old generation.
- System.gc() Triggered by JVM: Some JVM implementations may initiate garbage collection if the application explicitly requests memory and there isn’t enough available. This can happen in high memory usage situations.
- Application Idle Time: Some JVMs (like G1 Garbage Collector) may take advantage of idle CPU time to perform garbage collection in the background if configured to do so.
In most cases, you don’t need to manually manage or trigger garbage collection, as the JVM’s garbage collection algorithms are designed to efficiently manage memory usage.
The finalize()
method in Java was traditionally used as a way to perform cleanup operations on objects before they are removed by garbage collection. It is part of the java.lang.Object
class, and any class can override it to define actions that should take place just before an object is garbage-collected.
How finalize()
Works
When an object becomes eligible for garbage collection, the JVM checks if it has a finalize()
method. If it does, the JVM will call this method before collecting the object. The main intention was to allow the object to release resources it may be holding, such as closing files, releasing network connections, or cleaning up native resources.
Here’s a basic example of how finalize()
might look:
java
Copy code
@Override protected void finalize() throws Throwable { try { // Cleanup code (e.g., releasing resources) System.out.println("Object is being finalized and cleaned up."); } finally { super.finalize(); // Always call the superclass finalize } }
Limitations and Issues with finalize()
The finalize()
method has several drawbacks and is generally discouraged for a few reasons:
- Unpredictable Execution: There’s no guarantee when or if the
finalize()
method will be called. The JVM may delay garbage collection, meaning objects might stay in memory longer than necessary. - Performance Impact: Using
finalize()
can impact performance, as it introduces additional overhead. Garbage collection processes may need multiple cycles to fully clear objects with finalizers. - Reliability Issues: If the
finalize()
method throws an exception, it could prevent the object from being garbage-collected, leading to potential memory leaks. - Potential for Resurrection: Inside
finalize()
, it’s possible to make an object reachable again by assigningthis
to a global or static reference. This can lead to unexpected behavior and complicates garbage collection.
Better Alternatives to finalize()
Because of these limitations, finalize()
is deprecated starting from Java 9, and it is strongly discouraged in modern Java applications. Instead, the following alternatives are recommended:
- Try-With-Resources (Preferred for Auto-Closeable Resources):
- For resources like files, network connections, and database connections, Java provides the
try-with-resources
statement (from Java 7 onwards), which automatically closes resources when they go out of scope.
try (FileInputStream file = new FileInputStream("file.txt")) { // Use the file } catch (IOException e) { e.printStackTrace(); }
- For resources like files, network connections, and database connections, Java provides the
- Explicit Cleanup Methods:
- Implement an explicit
close()
method or other cleanup method to release resources, and ensure that users of your class call this method manually when done. - This pattern is often used with classes that implement
AutoCloseable
orCloseable
.
- Implement an explicit
- Cleaner API (Java 9 and Later):
- The
java.lang.ref.Cleaner
class offers a more flexible and efficient way to perform cleanup tasks. It lets you register cleanup actions for objects that can be invoked once they are no longer reachable, without the limitations offinalize()
.
Cleaner cleaner = Cleaner.create(); class Resource implements AutoCloseable { private final Cleaner.Cleanable cleanable; Resource() { this.cleanable = cleaner.register(this, () -> { System.out.println("Cleaning up resource."); }); } @Override public void close() { cleanable.clean(); } }
- The
Conclusion
The finalize()
method was originally designed for object cleanup before garbage collection, but it has limitations, including unpredictability and performance costs. Modern Java development favors alternatives like the try-with-resources statement, explicit cleanup methods, and the Cleaner
API for managing resources more safely and efficiently.
In Java, garbage collection (GC) is typically managed by a dedicated background thread or a set of threads within the Java Virtual Machine (JVM). These threads are part of the JVM’s internal management and work independently from the main application threads to perform memory management tasks without blocking the main execution flow.
Details of Garbage Collection Threads
- Dedicated Background Threads:
- The JVM spawns one or more background threads specifically for garbage collection. These threads are responsible for identifying unreachable objects, reclaiming memory, and performing cleanup as needed.
- The exact number and type of threads can vary based on the garbage collector chosen, JVM version, and configuration.
- Types of Garbage Collection Threads:
- Single-threaded GC (e.g., Serial GC): Uses a single garbage collection thread, suitable for smaller applications or single-CPU environments.
- Multi-threaded GC (e.g., Parallel GC, G1 GC): Uses multiple threads for garbage collection, making it better suited for applications that require high throughput and faster garbage collection cycles.
- Concurrent GC Threads (e.g., Concurrent Mark-Sweep (CMS), G1, ZGC): In collectors like CMS and G1, the GC threads perform some garbage collection work concurrently, meaning parts of the collection process happen alongside the application threads to reduce pause times.
- Daemon Threads:
- Garbage collection threads are often daemon threads, meaning they do not prevent the JVM from shutting down. When the main application finishes and no other non-daemon threads are running, the JVM can exit even if garbage collection threads are still active.
- Configuration Based on GC Algorithms:
- The JVM provides various garbage collection algorithms, each with its own thread management strategy:
- Serial GC (
-XX:+UseSerialGC
): Uses a single-threaded approach for garbage collection. - Parallel GC (
-XX:+UseParallelGC
): Uses multiple threads for stop-the-world (STW) phases, ideal for maximizing throughput in multi-core systems. - G1 GC (
-XX:+UseG1GC
): Uses multiple threads for concurrent marking and other phases, optimizing for predictable pause times. - ZGC and Shenandoah: Ultra-low-latency collectors designed to minimize pause times by using concurrent threads for most of the garbage collection work.
- Serial GC (
- The JVM provides various garbage collection algorithms, each with its own thread management strategy:
- Thread Prioritization:
- Garbage collection threads are typically low-priority threads compared to application threads, especially in collectors designed for minimal impact on application performance (like ZGC and Shenandoah).
- The JVM manages these threads’ priority and scheduling to balance garbage collection with the performance needs of the application.
Control Over GC Threads
Developers have limited control over garbage collection threads, but they can influence thread behavior and count via JVM flags, such as:
-XX:ParallelGCThreads=<N>
: Sets the number of threads for parallel garbage collectors.-XX:ConcGCThreads=<N>
: Controls the number of concurrent GC threads (used with G1 or CMS).
Summary
Garbage collection in Java is handled by dedicated GC threads managed by the JVM, with thread behavior and count depending on the chosen garbage collector. These threads are typically daemon threads that operate in the background, aiming to reclaim memory with minimal impact on the application’s performance. Different garbage collection algorithms have different threading strategies, balancing between throughput and low-latency requirements.
Overriding the finalize()
method is generally discouraged in modern Java applications due to its unpredictability and performance issues, and it has been deprecated since Java 9. However, there were some historical use cases where finalize()
was used to handle certain cleanup tasks before the garbage collector removed objects from memory. In such cases, developers aimed to ensure that resources were released if they hadn’t been explicitly freed.
Here are some of the use cases where finalize()
might have been overridden in the past:
1. Resource Cleanup for Native Resources (e.g., File Handles, Sockets)
If an object held native resources (such as file handles, sockets, or memory allocated outside the Java heap), finalize()
could be used as a fallback to release those resources. For example, if the application developer or user forgot to call a close()
method on an object, the finalize()
method would attempt to close it before the object was collected.
java
Copy code
@Override protected void finalize() throws Throwable { try { if (fileHandle != null) { fileHandle.close(); // Attempt to close file handle } } finally { super.finalize(); } }
2. Cleanup of Critical Resources in Case of Forgotten close()
Calls
In cases where objects implement Closeable
or AutoCloseable
(such as database connections, streams, or other I/O resources), finalize()
might have been overridden as a safety net to ensure that critical resources are released. This was particularly helpful in cases where a close()
or equivalent cleanup method was not called explicitly by the developer.
However, this is less of a concern with the try-with-resources
statement, which provides a more reliable way to close resources.
3. Releasing External Resources in Legacy Applications
In legacy applications, developers might not have had access to sophisticated resource management techniques (like try-with-resources
). They sometimes relied on finalize()
to clean up external resources, such as:
- Temporary files: Delete temporary files if they hadn’t been manually deleted.
- Cached data: Release memory allocated to cache-like structures to avoid memory leaks.
java
Copy code
@Override protected void finalize() throws Throwable { try { if (temporaryFile.exists()) { temporaryFile.delete(); } } finally { super.finalize(); } }
4. Logging or Debugging Object Lifecycle (for Development Purposes)
In certain debugging scenarios, developers might override finalize()
to log or trace object lifecycle events, especially to understand when objects were actually getting collected. While not practical in production, this could help in identifying memory leaks during development.
java
Copy code
@Override protected void finalize() throws Throwable { System.out.println("Object is being garbage collected: " + this); }
5. Releasing Resources in Low-Level Libraries
Low-level libraries, especially those dealing with hardware resources, sometimes used finalize()
as a safeguard for resource management. If a developer forgot to release hardware resources manually, finalize()
could act as a backup, ensuring that the resource was released when the object was garbage-collected.
Modern Alternatives to finalize()
For almost all of the above use cases, modern Java applications should avoid finalize()
and use alternative methods for resource management:
- Implementing
AutoCloseable
and Using Try-with-Resources: This ensures that resources are automatically released, makingfinalize()
unnecessary. - Using
Cleaner
API: Thejava.lang.ref.Cleaner
API, introduced in Java 9, provides a more flexible and reliable way to manage cleanup tasks without the drawbacks offinalize()
. - Explicit Cleanup Methods (
close()
): Adding an explicit cleanup method, such asclose()
, is still a best practice for releasing resources. Developers are encouraged to document the importance of calling these methods.
Conclusion
While finalize()
was historically used in a few scenarios for resource management, modern Java provides better alternatives. For any critical resource cleanup, try-with-resources
, AutoCloseable
, and the Cleaner
API are preferable to ensure predictable and efficient resource handling, without relying on the unreliable and deprecated finalize()
method.
An island of isolation is a situation in Java where a group of objects reference each other but have no external references from active parts of the application, making them unreachable and eligible for garbage collection. Even though they are mutually referenced, they can still be collected because there is no way for the application to reach any of these objects from active code.
Example of Island of Isolation
Consider the following example with two classes, ObjectA
and ObjectB
, where instances of each reference one another:
java
Copy code
class ObjectA { ObjectB b; public ObjectA(ObjectB b) { this.b = b; } } class ObjectB { ObjectA a; public ObjectB(ObjectA a) { this.a = a; } }
Now, if we create two objects, objA
and objB
, where objA
references objB
and objB
references objA
:
java
Copy code
public class IslandOfIsolationExample { public static void main(String[] args) { ObjectA objA = new ObjectA(null); ObjectB objB = new ObjectB(objA); // Creating circular reference objA.b = objB; // Breaking external references objA = null; objB = null; // At this point, objA and objB reference each other but are isolated. // They form an island of isolation. } }
Explanation
- Initially,
objA
referencesobjB
, andobjB
referencesobjA
. This creates a circular reference between the two objects. - When we set both
objA
andobjB
tonull
, the main method no longer has references to eitherobjA
orobjB
. - Since there are no external references to these objects from the application, they become unreachable.
- Island of Isolation: Even though
objA
andobjB
reference each other, there’s no way for the application to reach these objects. This “island” of mutually referencing objects is isolated from the rest of the application.
Garbage Collection and Island of Isolation
Java’s garbage collector can detect such isolated groups of objects and collect them, freeing up memory. The garbage collector uses algorithms to identify unreachable objects, and if an object or group of objects has no path from any active part of the application, it considers them eligible for garbage collection.
In this case, the objA
and objB
objects, although they reference each other, are isolated and will be collected during the next garbage collection cycle. This process helps in reclaiming memory that would otherwise remain occupied by objects that are no longer needed.