Maven
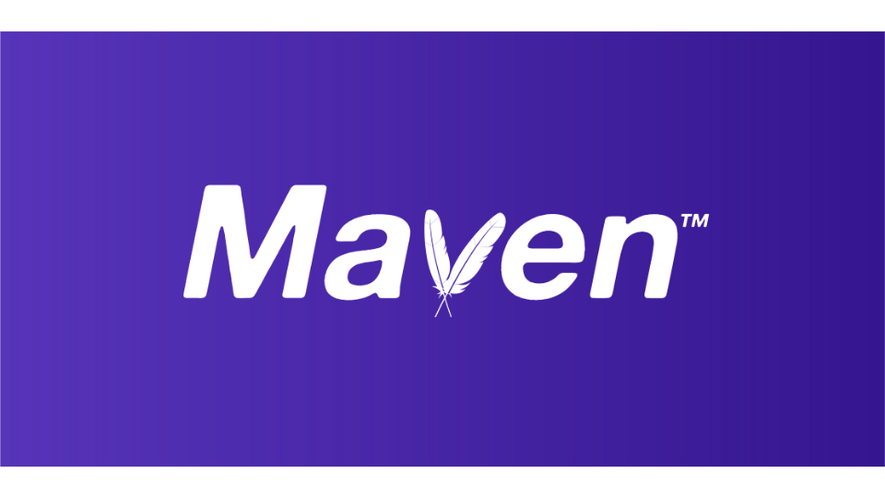
key components
pom,dependency,plugins etc
parent pom
the pom details that we will provide inside of <parent> tag in pom.xml
root pom
one which is in maven jar file which we download
xsd namespace information in pom.xml
…
most common tags in pom.xml
…
understanding how maven identify the application file or src directory and how it will place target folder by using root pom
…
profile in pom and build based on profile like test,production,stage etc
$mvn -P<profileid> package
$mvn -P<Profileid> clean
profile activation
groupId and artifactId
In Maven, groupId
and artifactId
are two fundamental coordinates that uniquely identify a project, along with the version.
groupId
- Purpose: The
groupId
represents the group or organization that the project belongs to. It is often structured like a domain name in reverse (e.g.,com.example
). - Uniqueness: The
groupId
should be unique to your organization or project to avoid conflicts with artifacts from other organizations. - Examples:
com.example
org.apache.maven.plugins
artifactId
- Purpose: The
artifactId
is the name of the specific project or module. It is a unique identifier within the group specified by thegroupId
. - Uniqueness: The combination of
groupId
andartifactId
should uniquely identify a single project or module. - Examples:
maven-plugin
spring-core
How They Work Together
- Combination: The combination of
groupId
andartifactId
uniquely identifies an artifact across all repositories. For example,com.example:my-app
uniquely identifies themy-app
project under thecom.example
group. - Versioning: When combined with the version, it fully qualifies the artifact (e.g.,
com.example:my-app:1.0.0
).
Example Structure
Here is an example of how groupId
and artifactId
are defined in a pom.xml
file:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0.0</version>
<dependencies>
<!-- Dependencies go here -->
</dependencies>
</project>
Summary
- groupId: Identifies the group or organization (e.g.,
com.example
). - artifactId: Identifies the specific project or module within the group (e.g.,
my-app
).
These identifiers help manage and organize dependencies and projects within Maven, ensuring that each artifact can be uniquely identified and retrieved.
mvn package and mvn install
The mvn package
and mvn install
commands are both part of the Maven build lifecycle, but they serve different purposes and are used in different scenarios:
mvn package
- Purpose: The
mvn package
command is used to compile your code and package it into a JAR, WAR, or another specified format. It executes all phases up topackage
phase. - Lifecycle Phases: This command runs the following lifecycle phases: validate, compile, test, and package.
- Output: The output of this command is the packaged file (JAR/WAR) in the
target
directory. - Use Case: Use
mvn package
when you want to compile your code and create the packaged artifact without installing it to the local repository. This is useful for quick builds, testing, or when you only need the artifact itself and don’t need it to be available for other projects to reference.
mvn install
- Purpose: The
mvn install
command packages the code (just likemvn package
) but also installs the packaged artifact into the local Maven repository. It executes all phases up toinstall
phase. - Lifecycle Phases: This command runs the following lifecycle phases: validate, compile, test, package, and install.
- Output: The output is the packaged file (JAR/WAR) in the
target
directory and a copy of this artifact in the local Maven repository (usually located in~/.m2/repository
). - Use Case: Use
mvn install
when you want to package your code and make the artifact available to other projects on your machine. This is useful in a multi-module project setup where different modules depend on each other, or when you are working on multiple related projects that need to reference each other’s artifacts.
Summary of Differences
- Lifecycle Phases:
mvn package
stops at thepackage
phase, whilemvn install
stops at theinstall
phase. - Artifact Handling:
mvn package
creates the artifact in thetarget
directory.mvn install
creates the artifact in thetarget
directory and also copies it to the local repository. - Use Cases:
mvn package
is for creating the artifact for testing or quick builds.mvn install
is for creating and installing the artifact so it can be used by other local projects.
Practical Example
- mvn package: You have a project that you want to build and package to run some tests or to deploy manually.
- mvn install: You have multiple projects, and Project B depends on Project A. You need to
mvn install
Project A so that Project B can find it in the local repository.
By understanding the differences and use cases, you can choose the appropriate command based on your specific needs during the Maven build process.
plugins and dependencies
In Maven, plugins
and dependencies
are two distinct concepts, each serving a different purpose in the build process.
Dependencies
- Purpose: Dependencies are external libraries or modules that your project needs to compile, run, or test. They are included in your project’s classpath.
- Scope: Dependencies can have different scopes (e.g.,
compile
,provided
,runtime
,test
,system
) that define how they are included in the build process. - Definition: Dependencies are defined in the
<dependencies>
section of thepom.xml
. - Example:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.9</version>
</dependency>
</dependencies>
Plugins
- Purpose: Plugins extend Maven’s functionality. They define tasks that can be executed during the build process (e.g., compiling code, running tests, packaging, deploying).
- Scope: Plugins can be bound to specific phases of the Maven build lifecycle (e.g.,
compile
,test
,package
,install
,deploy
). - Definition: Plugins are defined in the
<build><plugins>
section of thepom.xml
. - Example:
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<excludes>
<exclude>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</exclude>
</excludes>
</configuration>
</plugin>
</plugins>
</build>
Key Differences
- Functionality:
- Dependencies: Provide external libraries your project needs to function.
- Plugins: Provide additional functionality to the build process, such as compiling code, packaging the application, running tests, and more.
- Usage:
- Dependencies: Used within the project code (e.g., you import classes from dependencies).
- Plugins: Used to perform build tasks (e.g., compiling, packaging) and are not directly referenced in the project code.
- Configuration Location:
- Dependencies: Defined in the
<dependencies>
section. - Plugins: Defined in the
<build><plugins>
section.
- Lifecycle:
- Dependencies: Managed and resolved during the build process to ensure all necessary libraries are available.
- Plugins: Executed at specific phases of the Maven build lifecycle to perform tasks.
Example Use Cases
- Dependencies: You include a logging library like Log4j in your project to handle logging.
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.14.1</version>
</dependency>
- Plugins: You use the Maven Compiler Plugin to specify the Java version for compiling your project.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
Understanding these differences helps in correctly setting up and managing your Maven project for successful builds and dependency management.
Note : One of the best example for Plugin is the spring-boot-maven-plugin
is a Maven plugin provided by the Spring Boot framework. It simplifies the process of creating executable JARs or WARs, running Spring Boot applications, and managing dependencies for Spring Boot projects.
Features
- Build Executable JAR/WAR: Packages Spring Boot applications into executable JAR or WAR files, allowing them to be run as standalone applications.
- Run Spring Boot Applications: Provides goals to run Spring Boot applications directly from Maven.
- Repackage Existing JARs/WARs: Repackages existing JARs/WARs to make them executable.
- Dependency Management: Ensures that dependencies are included correctly in the final package.
Use Cases
- Development: Use
spring-boot:run
to quickly start your application during development.- $
mvn spring-boot:run
- $
- Packaging: Use
spring-boot:repackage
to create an executable JAR/WAR file for deployment.- $
mvn clean package
- $
- Deployment: Deploy the generated JAR/WAR file to a server or cloud environment. The application can be started with:
- $
java -jar target/my-spring-boot-app-1.0.0.jar
- $
transitive dependency
In Maven, a transitive dependency is a dependency that is not directly included in your project’s dependencies but is included through another dependency. This means if your project depends on library A, and library A depends on library B, then your project will also have a dependency on library B. Maven automatically resolves these transitive dependencies and includes them in your project’s build.
Understanding by the spring-boot-starter-web
Example
Direct Dependency
- Spring boot project directly depends on
spring-boot-starter-web
.
Transitive Dependencies
spring-boot-starter-web
might depend onspring-boot-starter
,spring-web
, andspring-webmvc
.spring-boot-starter
might further depend onspring-core
,spring-context
, etc.spring-webmvc
might depend onspring-beans
,spring-aop
, etc.
settings.xml in maven folder/config that we downloaded
… we can configure the maven repositories path based on profile by using settings.xml file to download the dependencies based on profile. and we can configure other things also using settings.xml file
Dependency Scope
In Maven, the <scope>
element is used to specify the visibility and availability of a dependency in various build phases and classpaths (compile, test, runtime, etc.). The scope determines how the dependency is treated and where it is included in the build lifecycle.
Common Scopes
compile
- Default Scope: If no scope is specified,
compile
is assumed. - Availability: The dependency is available in all classpaths (compilation, runtime, and test).
- Use Case: Libraries that are required for the project to compile and run.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
<scope>compile</scope>
</dependency>
provided
- Availability: The dependency is available in the compilation and test classpaths but not in the runtime classpath.
- Use Case: Libraries that are provided by the runtime environment or container (e.g., servlet APIs provided by the application server).
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
runtime
- Availability: The dependency is not available in the compilation classpath but is available in the runtime and test classpaths.
- Use Case: Libraries that are not needed for compilation but are required for execution (e.g., JDBC drivers).
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
<scope>runtime</scope>
</dependency>
test
- Availability: The dependency is only available in the test classpath.
- Use Case: Libraries needed only for testing purposes (e.g., JUnit, Mockito).
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
system
- Availability: Similar to
provided
, but you have to explicitly provide the JAR which is not managed by Maven repositories. ThesystemPath
element must be specified. - Use Case: Libraries that are provided locally and not available in Maven repositories.
- Note: Using
system
scope is generally discouraged as it breaks the dependency management and reproducibility provided by Maven.
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
<scope>system</scope>
<systemPath>${project.basedir}/lib/my-library.jar</systemPath>
</dependency>
import
- Usage: Used only in the
<dependencyManagement>
section to import dependencies from a BOM (Bill of Materials). - Use Case: Importing a list of dependencies defined in another POM, typically used with platform projects like Spring Boot.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.4</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
Example pom.xml
with Different Scopes
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-app</artifactId>
<version>1.0.0</version>
<dependencies>
<!-- Compile scope dependency -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
<scope>compile</scope>
</dependency>
<!-- Provided scope dependency -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
<!-- Runtime scope dependency -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
<scope>runtime</scope>
</dependency>
<!-- Test scope dependency -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Summary
- Compile: Default scope, available in all classpaths.
- Provided: Available in compile and test classpaths, but not at runtime.
- Runtime: Available in runtime and test classpaths, but not during compile.
- Test: Only available in the test classpath.
- System: Similar to provided, but requires an explicit path to the JAR file.
- Import: Used to import dependencies from a BOM in dependency management.